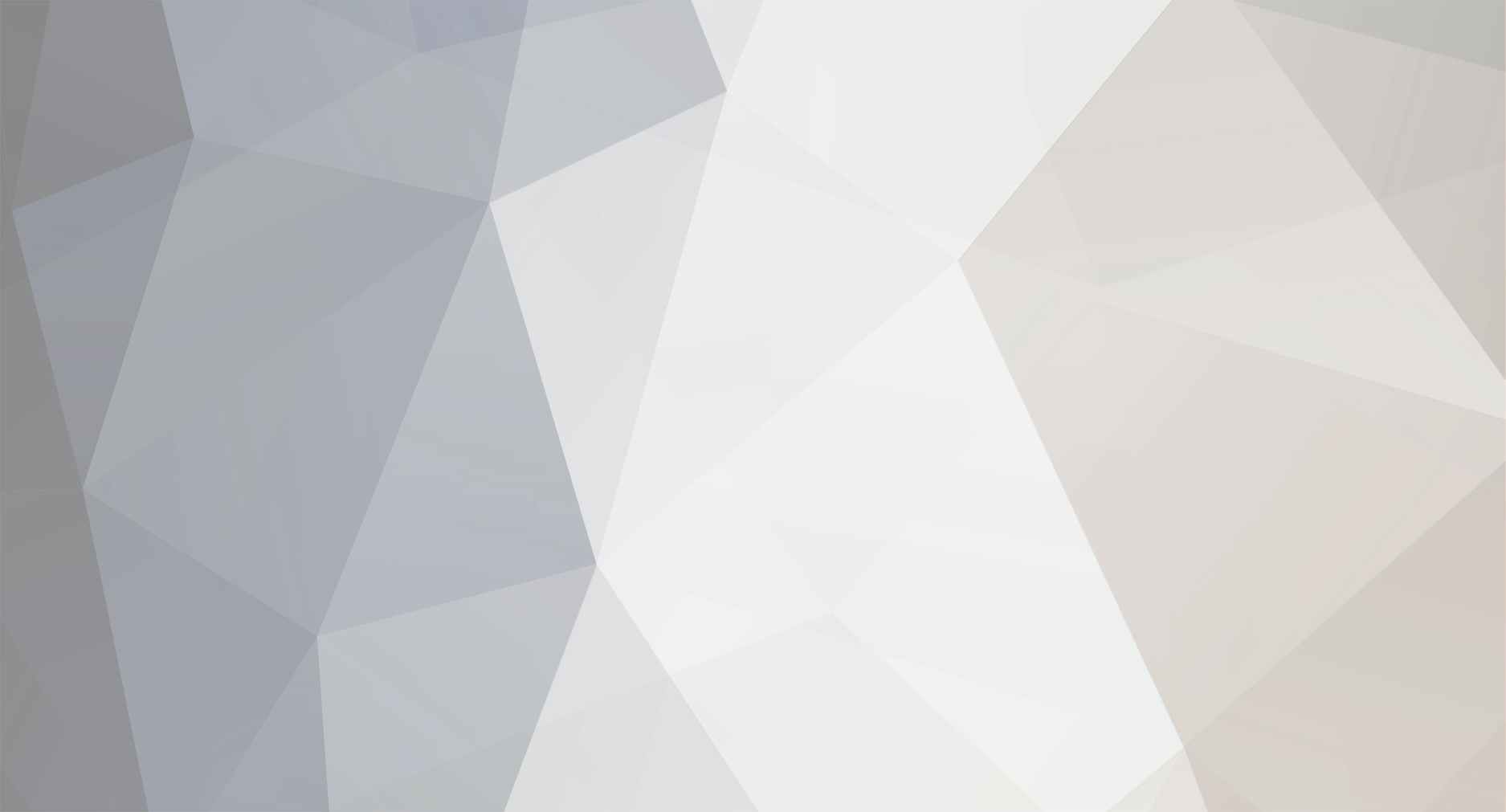
thieled
Members-
Gesamte Inhalte
6 -
Benutzer seit
-
Letzter Besuch
thieled's Achievements
Newbie (1/14)
0
Reputation in der Community
-
Well I've edited the xively code for beebotte as follows: #!/usr/bin/env python # -*- coding: utf-8 -*- import socket import sys import time import math import logging as log log.basicConfig(level=log.INFO) from beebotte import * from tinkerforge.ip_connection import IPConnection from tinkerforge.ip_connection import Error from tinkerforge.brick_master import Master from tinkerforge.bricklet_lcd_20x4 import LCD20x4 from tinkerforge.bricklet_ambient_light import AmbientLight from tinkerforge.bricklet_humidity import Humidity from tinkerforge.bricklet_barometer import Barometer class WeatherStation: HOST = "localhost" PORT = 4223 ipcon = None lcd = None al = None hum = None baro = None def __init__(self): # beebotte.com parameters AccessKey='...' SecurityKey='...' # connect to beebotte.com self.bbt = BBT(AccessKey,SecurityKey) self.temp_resource=Resource(self.bbt,'RaspberryPiWeatherStation','Temperature') self.humid_resource=Resource(self.bbt,'RaspberryPiWeatherStation','Humidity') self.airpress_resource=Resource(self.bbt,'RaspberryPiWeatherStation','AirPressure') self.amblight_resource=Resource(self.bbt,'RaspberryPiWeatherStation','AmbientLight') print('beebotte connected') self.ipcon = IPConnection() while True: try: self.ipcon.connect(WeatherStation.HOST, WeatherStation.PORT) break except Error as e: log.error('Connection Error: ' + str(e.description)) time.sleep(1) except socket.error as e: log.error('Socket error: ' + str(e)) time.sleep(1) self.ipcon.register_callback(IPConnection.CALLBACK_ENUMERATE, self.cb_enumerate) self.ipcon.register_callback(IPConnection.CALLBACK_CONNECTED, self.cb_connected) while True: try: self.ipcon.enumerate() break except Error as e: log.error('Enumerate Error: ' + str(e.description)) time.sleep(1) def cb_illuminance(self, illuminance): global amblight_resource if self.lcd is not None: text = 'Illuminanc %6.2f lx' % (illuminance/10.0) self.lcd.write_line(0, 0, text) self.amblight_resource.write(illuminance/10.0) log.info('Write to line 0: ' + text) def cb_humidity(self, humidity): global humid_resource if self.lcd is not None: text = 'Humidity %6.2f %%' % (humidity/10.0) self.lcd.write_line(1, 0, text) self.humid_resource.write(humidity/10.0) log.info('Write to line 1: ' + text) def cb_air_pressure(self, air_pressure): global airpress_resource global temp_resource if self.lcd is not None: text = 'Air Press %7.2f mb' % (air_pressure/1000.0) self.lcd.write_line(2, 0, text) self.airpress_resource.write(air_pressure/1000.0) log.info('Write to line 2: ' + text) try: temperature = self.baro.get_chip_temperature()/100.0 except Error as e: log.error('Could not get temperature: ' + str(e.description)) return # \xDF == ° on LCD 20x4 charset text = 'Temperature %5.2f \xDFC' % temperature self.lcd.write_line(3, 0, text) self.temp_resource.write(temperature) log.info('Write to line 3: ' + text.replace('\xDF', '°')) def cb_enumerate(self, uid, connected_uid, position, hardware_version, firmware_version, device_identifier, enumeration_type): if enumeration_type == IPConnection.ENUMERATION_TYPE_CONNECTED or \ enumeration_type == IPConnection.ENUMERATION_TYPE_AVAILABLE: if device_identifier == LCD20x4.DEVICE_IDENTIFIER: try: self.lcd = LCD20x4(uid, self.ipcon) self.lcd.clear_display() self.lcd.backlight_on() log.info('LCD20x4 initialized') except Error as e: log.error('LCD20x4 init failed: ' + str(e.description)) self.lcd = None elif device_identifier == AmbientLight.DEVICE_IDENTIFIER: try: self.al = AmbientLight(uid, self.ipcon) self.al.set_illuminance_callback_period(10000) self.al.register_callback(self.al.CALLBACK_ILLUMINANCE, self.cb_illuminance) log.info('AmbientLight initialized') except Error as e: log.error('AmbientLight init failed: ' + str(e.description)) self.al = None elif device_identifier == Humidity.DEVICE_IDENTIFIER: try: self.hum = Humidity(uid, self.ipcon) self.hum.set_humidity_callback_period(10000) self.hum.register_callback(self.hum.CALLBACK_HUMIDITY, self.cb_humidity) log.info('Humidity initialized') except Error as e: log.error('Humidity init failed: ' + str(e.description)) self.hum = None elif device_identifier == Barometer.DEVICE_IDENTIFIER: try: self.baro = Barometer(uid, self.ipcon) self.baro.set_air_pressure_callback_period(10000) self.baro.register_callback(self.baro.CALLBACK_AIR_PRESSURE, self.cb_air_pressure) log.info('Barometer initialized') except Error as e: log.error('Barometer init failed: ' + str(e.description)) self.baro = None def cb_connected(self, connected_reason): if connected_reason == IPConnection.CONNECT_REASON_AUTO_RECONNECT: log.info('Auto Reconnect') while True: try: self.ipcon.enumerate() break except Error as e: log.error('Enumerate Error: ' + str(e.description)) time.sleep(1) if __name__ == "__main__": log.info('Weather Station: Start') weather_station = WeatherStation() if sys.version_info < (3, 0): input = raw_input # Compatibility for Python 2.x input('Press key to exit\n') if weather_station.ipcon != None: weather_station.ipcon.disconnect() log.info('Weather Station: End') It runs OK for a while and then I get the following error message: Exception in thread Callback-Processor: Traceback (most recent call last): File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connectionpool.py", line 518, in urlopen body=body, headers=headers) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connectionpool.py", line 330, in _make_request conn.request(method, url, **httplib_request_kw) File "/usr/lib/python3.2/http/client.py", line 970, in request self._send_request(method, url, body, headers) File "/usr/lib/python3.2/http/client.py", line 1008, in _send_request self.endheaders(body) File "/usr/lib/python3.2/http/client.py", line 966, in endheaders self._send_output(message_body) File "/usr/lib/python3.2/http/client.py", line 811, in _send_output self.send(msg) File "/usr/lib/python3.2/http/client.py", line 749, in send self.connect() File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connection.py", line 155, in connect conn = self._new_conn() File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connection.py", line 134, in _new_conn (self.host, self.port), self.timeout, **extra_kw) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/util/connection.py", line 64, in create_connection for res in socket.getaddrinfo(host, port, 0, socket.SOCK_STREAM): socket.gaierror: [Errno -2] Name or service not known During handling of the above exception, another exception occurred: Traceback (most recent call last): File "/usr/local/lib/python3.2/dist-packages/requests/adapters.py", line 370, in send timeout=timeout File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connectionpool.py", line 564, in urlopen _pool=self, _stacktrace=stacktrace) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/util/retry.py", line 245, in increment raise six.reraise(type(error), error, _stacktrace) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/packages/six.py", line 309, in reraise raise value.with_traceback(tb) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connectionpool.py", line 518, in urlopen body=body, headers=headers) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connectionpool.py", line 330, in _make_request conn.request(method, url, **httplib_request_kw) File "/usr/lib/python3.2/http/client.py", line 970, in request self._send_request(method, url, body, headers) File "/usr/lib/python3.2/http/client.py", line 1008, in _send_request self.endheaders(body) File "/usr/lib/python3.2/http/client.py", line 966, in endheaders self._send_output(message_body) File "/usr/lib/python3.2/http/client.py", line 811, in _send_output self.send(msg) File "/usr/lib/python3.2/http/client.py", line 749, in send self.connect() File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connection.py", line 155, in connect conn = self._new_conn() File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/connection.py", line 134, in _new_conn (self.host, self.port), self.timeout, **extra_kw) File "/usr/local/lib/python3.2/dist-packages/requests/packages/urllib3/util/connection.py", line 64, in create_connection for res in socket.getaddrinfo(host, port, 0, socket.SOCK_STREAM): requests.packages.urllib3.exceptions.ProtocolError: ('Connection aborted.', gaierror(-2, 'Name or service not known')) During handling of the above exception, another exception occurred: Traceback (most recent call last): File "/usr/lib/python3.2/threading.py", line 740, in _bootstrap_inner self.run() File "/usr/lib/python3.2/threading.py", line 693, in run self._target(*self._args, **self._kwargs) File "/home/pi/weather_station/tinkerforge/ip_connection.py", line 812, in callback_loop self.dispatch_packet(data) File "/home/pi/weather_station/tinkerforge/ip_connection.py", line 793, in dispatch_packet cb(self.deserialize_data(payload, form)) File "beebotte.py", line 88, in cb_humidity self.humid_resource.write(humidity/10.0) File "/home/pi/weather_station/beebotte/__init__.py", line 428, in write return self.bbt.write(self.channel, self.resource, ts = ts, data = data) File "/home/pi/weather_station/beebotte/__init__.py", line 287, in write response = self.__postData__( endpoint, json.dumps(body, separators=(',', ':')), True ) File "/home/pi/weather_station/beebotte/__init__.py", line 169, in __postData__ r = requests.post( url, data=data, headers=headers ) File "/usr/local/lib/python3.2/dist-packages/requests/api.py", line 99, in post return request('post', url, data=data, json=json, **kwargs) File "/usr/local/lib/python3.2/dist-packages/requests/api.py", line 49, in request response = session.request(method=method, url=url, **kwargs) File "/usr/local/lib/python3.2/dist-packages/requests/sessions.py", line 461, in request resp = self.send(prep, **send_kwargs) File "/usr/local/lib/python3.2/dist-packages/requests/sessions.py", line 573, in send r = adapter.send(request, **kwargs) File "/usr/local/lib/python3.2/dist-packages/requests/adapters.py", line 415, in send raise ConnectionError(err, request=request) requests.exceptions.ConnectionError: ('Connection aborted.', gaierror(-2, 'Name or service not known')) Any advice would be much appreciated!
-
Thanks nattfoedd - I'll look into it. However as a newbie programmer I suspect I'm not quite at the level to implement this myself. Has anyone written python code that interacts with this site that I could look at?
-
I've built the weather station and wanted to post my data to a website. Tinkerforge have python code to do this using xively. However when I try to create an xively account I've been put on a waiting list. Is there any alternative that a newbie pythoner would be able to implement? Many thanks!
-
Problem solved - many thanks borg!
-
Here's the code ... #!/usr/bin/env python # -*- coding: utf -8 -*- HOST="localhost" PORT=4223 UID="ogH" import time; from tinkerforge.ip_connection import IPConnection from tinkerforge.bricklet_lcd_20x4 import LCD20x4 # Callback functions for button status def cb_pressed(i): print('Pressed: '+ str(i)) BacklightON = lcd.is_backlight_on() print(BacklightON) if __name__ == "__main__": ipcon=IPConnection() # Create IP connection lcd=LCD20x4(UID,ipcon) # Create device object ipcon.connect(HOST,PORT) # Connect to brickd # Don't use device before ipcon is connected # Register button status callback to cb_pressed lcd.register_callback(lcd.CALLBACK_BUTTON_PRESSED,cb_pressed) # lcd.backlight_off() BacklightON = lcd.is_backlight_on() print(BacklightON) while True: n=0 # Line 0 Line="Button 0: MAIN MENU" # Determine start position to be centred # Position=(20-len(Line))/2 Position=0 # Write Line 0 lcd.write_line(0,Position,Line) # Line 1 Line="Button 1: DATE/TIME" # Determine start position to be centred # Position=(20-len(Line))/2 # Write Line 1 lcd.write_line(1,Position,Line) # Line 2 Line="Button 2: WEATHER" # Determine start position to be centred # Position=(20-len(Line))/2 # Write Line 2 lcd.write_line(2,Position,Line) and here's the error message ... >>> False Pressed: 0 Exception in thread Callback-Processor: Traceback (most recent call last): File "/home/pi/weather_station/tinkerforge/ip_connection.py", line 938, in send_request response = device.response_queue.get(True, self.timeout) File "/usr/lib/python3.2/queue.py", line 193, in get raise Empty queue.Empty During handling of the above exception, another exception occurred: Traceback (most recent call last): File "/usr/lib/python3.2/threading.py", line 740, in _bootstrap_inner self.run() File "/usr/lib/python3.2/threading.py", line 693, in run self._target(*self._args, **self._kwargs) File "/home/pi/weather_station/tinkerforge/ip_connection.py", line 812, in callback_loop self.dispatch_packet(data) File "/home/pi/weather_station/tinkerforge/ip_connection.py", line 793, in dispatch_packet cb(self.deserialize_data(payload, form)) File "/home/pi/weather_station/test_lcd.py", line 17, in cb_pressed BacklightON = lcd.is_backlight_on() File "/home/pi/weather_station/tinkerforge/bricklet_lcd_20x4.py", line 123, in is_backlight_on return self.ipcon.send_request(self, BrickletLCD20x4.FUNCTION_IS_BACKLIGHT_ON, (), '', '?') File "/home/pi/weather_station/tinkerforge/ip_connection.py", line 947, in send_request raise Error(Error.TIMEOUT, msg) tinkerforge.ip_connection.Error: -1: Did not receive response for function 5 in time Many thanks borg.
-
A very new python programmer here who has built the Tinkerforge weather station connected to a Raspberry Pi. I am starting to tinker with the Tinkerforge code and want to implement the is_backlight_on function for the LCD. I more often than not get a timeout error when I call this function; sometimes it works but mostly not. Any help would be appreciated for a newbie!