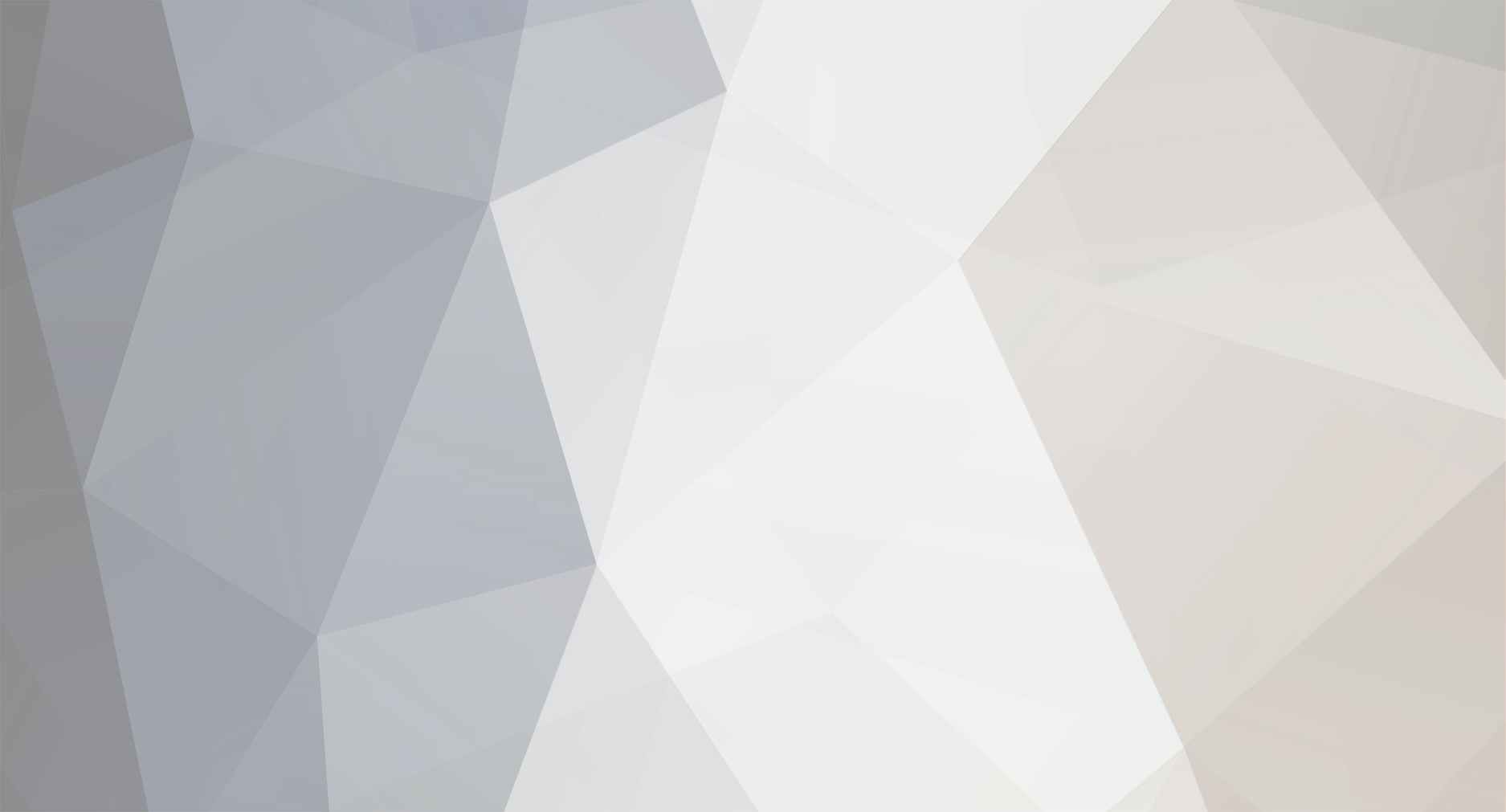
photron
-
Gesamte Inhalte
3.216 -
Benutzer seit
-
Letzter Besuch
-
Tagessiege
57
Posts erstellt von photron
-
-
Sehr gut, dann wird die Fronius Speicherunterstützung Teil des nächsten Firmware Release sein.
Auto SOC auslesen benötigt ISO 15118, das benötigt einen größeren Schwung extra Hardware, die die Wallbox leider nicht hat.
Du kannst den Umweg über die Cloud des Autoherstellers gehen um an den Auto SOC zu kommen. Das hat aber auch so seine Tücken. Das kannst du mit EVCC bewerkstelligen.
-
1
-
-
Bitte die angehängte Firmware testen. Ich bin zuversichtlich dass es jetzt funktioniert.
Edit: Angehängte Firmware gelöscht. Diese Funktion ist schon Teil der offiziellen Firmware.
-
1
-
-
Okay, danke. Ich glaube ich habe die Verwirrung gelöst. Ich habe in Gen24_Primo_Symo_Inverter_Register_Map_Float_storage.xlsx geguckt. Dort sind die Register die uns interesieren um +10 verschoben, bedingt dadurch das einige Werte vorher in zwei statt in einem Register abgebildet werden. Dann kommt hinzu, dass im Dokument Registernummern (1-basiert) und nicht Registeraddressen (0-basiert) angegeben sind. Ich bestätige, ich komme jetzt auch auf Registeradresse 40351 für State of Charge ist.
-
Die Werte sind ja mal völlig daneben. Ich denke die Dokumentation über die Registermap die ich verwendet habe passt nicht.
@pene8 Woher hast du dass Register 40351 State of Charge ist? Das ist laut Dokumentation die ich habe etwas völlig anderes.
-
Interessant. Das ChaState_SF darf wohl nicht gelsen werden. Dort steht der Skalierfaktor für den ChaState Wert (State of Charge) des Speichers drin. Die Dokumentation sagt, dass der fest -2 (also 0,01) ist. Ich habe aber versucht den Wert trotzdem zu lesen. Das habe ich jetzt geändert und nehme fest -2 an.
Bitte die angehängte Firmware testen. Die Einstellungen können so belassen werden. Nur die Firmware fachen und schauen, ob es dann geht.
EDIT: Fehlerhafte Firmware gelöscht, neue Firmware in meinem nächsten Post.
-
Fronius meldet die Speicherwerte nicht direkt über SunSpec, sondern missbraucht dafür MPP Tracker 3 und 4 im SunSpec Modell 160.
Für den Moment hier erstmal eine Firmware mit Support für den Fronius GEN24 Plus Speicher. Das ist absolut ungetestet. Im schlimmsten Fall funktioniert es einfach nicht.
Vor dem Update die Batteriespeicher-Option auf "Kein Batteriespeicher" stellen und speichern, soll ich von MatzeTF ausrichten. Danach dann den neuen Zähler einrichten und auswählen.
Für den neuen Zähler als Klasse Modbus/TCP wählen, Anzeigename und Host eintragen, Port auf 502 belassen, als Registertabelle Fronius GEN24 Plus Hybrid-Wechselrichter wählen, als Virtueller Zähler Speicher wählen und Geräteadresse auf 1 belassen. Dann Hinzufügen, Speichern und Neustarten klicken. Im besten Fall funktioniert das einfach so und die Messwerte für den Speicher werden angezeigt.
EDIT: Fehlerhafte Firmware gelöscht, neue Firmware in meinem nächsten Post.
-
@TobiSt Teste bitte die angehängte Version.
-
Schau bitte mal, ob du die neuste Firmware Version 2.0.4 auf dem HAT Brick hast, und ob du die neuste brickd Version 2.4.7 laufen hast.
-
Laut Datenblatt ist der E38S6G5-600B-G24N ein Quadraturencoder, der mit bis zu 24V arbeiten kann. Dafür ist unser Industrial Counter Bricklet bestens geeignet:
-
On 9/17/2024 at 4:13 PM, nineax said:
I get the message “Brick Daemon 2.4.7 stopped”
Where do you get this message?
Could you please show the full output of the command "systemctl status brickd", the command "uname -a", the command "gpioinfo" and attach the /var/log/brickd.log file from your Raspberry Pi?
-
Euler angles are problematic, as you already found out. The IMU Bricklet also provides the oriantation in form of a quaternion and Wikipedia explains how to convert a quaternion into a rotation matrix:
https://en.wikipedia.org/wiki/Quaternions_and_spatial_rotation#Quaternion-derived_rotation_matrix
-
Wir haben das bei @COW getestet und es gab bis einschlieslich WARP2 Firmware 2.4.1 einen Fehler in der Prüfung, der dazu geführt hat, dass die Prüfung als okay betrachtet wurde und dann die Firmware eingespielt wurde, obwohl es nicht erlaubt gewesen wäre.
Diese Problem ist mit den aktuellen Firmware-Version (u.a. WARP2 Firmware 2.5.0) behoben und Firmware-Updates werden im Falle eines angeschlossenen Fahrzeugs zuverlässig verweigert.
-
1
-
-
Mit welchem Browser hast du das hinbeommen?
On 9/3/2024 at 7:13 AM, COW said:Kann ich auch eine Box wieder auf 2.4.1 zurücksetzen? Dann könnte ich ein Video davon machen.
Ja, das ist kein Problem.
-
Ich habe das gerade getestet und es funktoniert wie es soll in Chrome 128 und Firefox 129 auf Linux. Auf der Firmware-Aktualisierung-Seite wird die manuelle Aktualisierung verweigert, wenn ein Auto angeschlossen ist. Ich kann das klicken was ich will.
Auf der Recovery-Seite darf man flashen auch wenn Auto angeschlossen ist. Über die Recovery-Seite reden wir hier aber nicht, richtig?
@COW und @wuesten_fuchs: Könnt ihr mir bitte einmal genauer erklären was ihr tut, um die Prüfung zu umgehen?
-
Sorry, das soll so nicht funktionieren, das ist ein Bug.
Von welcher Firmware-Version aus hast du das Update gemacht? Also welche Fmware-Version hat dieses Verhalten zugelassen?
-
Ohne Vorzeichen muss du "Bezug plus Einspeisung" wählen. Darauf kann das dynamische Lastmanagement abr nicht regeln. Wir brauchen den gerichteten Phasenstrom. Kann der Zähler andere Werte liefern an denen ersichtlich ist, ob gerade bezogen oder eingespeist wird?
-
Im Moment gibt es da noch nichts für. Das ist ein offenes TODO: https://github.com/Tinkerforge/esp32-firmware/issues/47
-
Die Sortierung in beiden Screenshots ist richtig. Du hast im ersten Screenshot die Sortigung auf "nach Position absteigend" gestellt und im zweiten auf "nach Position aufsteigend". Falls das nicht absi chtlich ist, dann hast du aus Versehen auf den "Position" Eintrag geklickt und dadurch die Sortierung umgestellt.
Bleibt die Frage, warum der 3.2er Master Brick oben auf dem Stack seine Bricklets nicht meldet. Kannst du einmal zeigen wie der funktionierende Fall mit Master Brick 3.2 alleine oder unten im Stack in Brick Viewer aussieht?
-
brickd 2.4.7 is now available from our APT server.
-
1
-
-
brickd 2.4.7 is now available from our APT server.
-
-
-
On 7/22/2024 at 2:59 PM, jannis said:
Im Brick Viewer kann ich beide Geräte sehen und die Stromstärke am Bricklet anzeigen lassen.
Dafür mustest du im Brick Viewer localhost zum Hostnamen oder der IP-Adresse des ESP32 Ethernet Brick ändern. Das musst du auch beim Aufruf von tinkerforge_mqtt machen. Du hast dort --ipcon-host localhost stehen, dort musst du localhost auch zum Hostnamen oder der IP-Adresse des ESP32 Ethernet Brick ändern.
-
Please try the attached brickd version:
sudo dpkg -i brickd_2.4.7_armhf.deb
Java IPConnection.DisconnectedListener funktioniert nicht?
in Software, Programmierung und externe Tools
Geschrieben
Das ist leider so erstmal absolut erwartetes Verhalten. Eine TCP/IP Verbindung wird nicht passive getrennt. Die Verbindung besteht so lange bis eine der beiden Seiten aktive die Verbinfung schließt. Trennen des Netzwerkkabels oder Abschalten einer Seite führt nicht dazu, dass die andere Seite die Verbindung für geschlossen hält. Dieser Fall lässt sich nur durch einen aktiven Heartbeat-Mechanismus lösen, den keines unserer API Bindings bisher untersützt, sorry.
Die TCP/IP Verbindung kann erst als unterbrochen durch die API Bindings erkannt werden, wenn du nach dem Trennen der Stromversorgung die Stromversorgung wieder anschließt. Denn dann ist die Verbindung auf der Gegenseite geschlossen und die Gegenseite antwortet mit einem TCP/IP Reset auf eingehende Pakete. Vorher laufen die Pakete einfach ins Leere, was erlaubt ist in TCP/IP.
Ich kann allerdings nicht nachstellen, dass Connected-Listener oder Autoreconnect notwendig wären. Folgendes Beispiel funktioniert, mit den beschriebenen Einschränkungen.